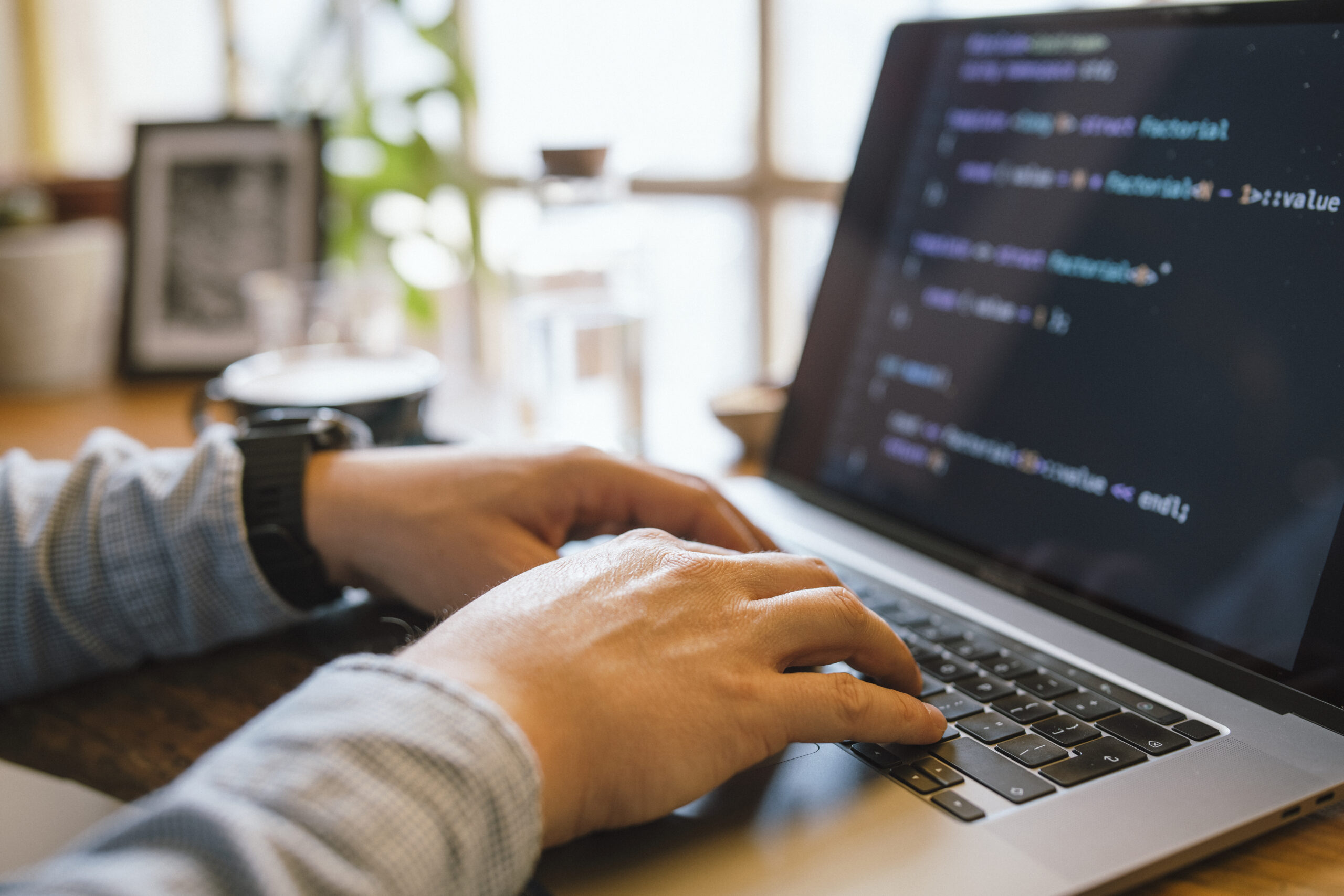
Debugging is one of the most vital — nonetheless frequently ignored — expertise in the developer’s toolkit. It isn't really just about fixing broken code; it’s about knowledge how and why matters go Completely wrong, and learning to Believe methodically to solve issues successfully. Irrespective of whether you are a starter or simply a seasoned developer, sharpening your debugging skills can save hrs of disappointment and substantially increase your productiveness. Allow me to share many strategies to help builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
One of several quickest methods developers can elevate their debugging competencies is by mastering the instruments they use every single day. When producing code is one particular A part of development, recognizing tips on how to communicate with it properly in the course of execution is equally significant. Present day improvement environments occur equipped with impressive debugging capabilities — but numerous builders only scratch the surface area of what these tools can do.
Acquire, by way of example, an Integrated Progress Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments help you set breakpoints, inspect the worth of variables at runtime, stage through code line by line, and in many cases modify code around the fly. When utilized the right way, they Allow you to notice specifically how your code behaves during execution, that's priceless for monitoring down elusive bugs.
Browser developer resources, which include Chrome DevTools, are indispensable for front-conclusion developers. They enable you to inspect the DOM, watch network requests, look at real-time functionality metrics, and debug JavaScript in the browser. Mastering the console, sources, and network tabs can switch disheartening UI concerns into workable responsibilities.
For backend or program-stage developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer deep Handle around operating procedures and memory management. Finding out these instruments may have a steeper Understanding curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, turn out to be relaxed with Variation Manage techniques like Git to be aware of code record, find the precise instant bugs were introduced, and isolate problematic adjustments.
Eventually, mastering your instruments indicates going over and above default options and shortcuts — it’s about establishing an personal familiarity with your progress setting making sure that when challenges crop up, you’re not shed in the dark. The better you know your equipment, the more time it is possible to devote fixing the actual issue as opposed to fumbling by the procedure.
Reproduce the situation
Among the most important — and sometimes neglected — measures in productive debugging is reproducing the situation. Ahead of jumping in to the code or creating guesses, builders will need to produce a reliable setting or situation exactly where the bug reliably seems. Devoid of reproducibility, repairing a bug turns into a sport of chance, normally resulting in wasted time and fragile code modifications.
The initial step in reproducing a dilemma is collecting as much context as feasible. Check with queries like: What steps brought about The problem? Which atmosphere was it in — development, staging, or manufacturing? Are there any logs, screenshots, or error messages? The greater depth you might have, the much easier it gets to isolate the precise situations under which the bug takes place.
When you finally’ve collected plenty of data, make an effort to recreate the issue in your neighborhood environment. This may mean inputting the identical info, simulating equivalent user interactions, or mimicking system states. If The difficulty appears intermittently, think about crafting automated checks that replicate the edge conditions or condition transitions included. These assessments not merely help expose the challenge but also protect against regressions in the future.
From time to time, the issue might be ecosystem-particular — it would materialize only on particular running units, browsers, or underneath particular configurations. Making use of instruments like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms is often instrumental in replicating this sort of bugs.
Reproducing the situation isn’t simply a step — it’s a attitude. It calls for endurance, observation, in addition to a methodical method. But as you can consistently recreate the bug, you are presently midway to repairing it. That has a reproducible scenario, You should use your debugging applications more successfully, check likely fixes safely and securely, and converse additional Obviously using your staff or people. It turns an summary grievance into a concrete challenge — and that’s the place developers thrive.
Go through and Realize the Error Messages
Error messages are often the most respected clues a developer has when anything goes Mistaken. As an alternative to observing them as annoying interruptions, developers ought to discover to deal with error messages as immediate communications with the technique. They typically inform you just what happened, in which it occurred, and occasionally even why it transpired — if you know the way to interpret them.
Start off by reading through the message diligently and in comprehensive. Quite a few developers, specially when underneath time stress, look at the 1st line and straight away start out producing assumptions. But deeper from the error stack or logs may perhaps lie the real root trigger. Don’t just copy and paste mistake messages into engines like google — study and have an understanding of them 1st.
Break the error down into components. Is it a syntax mistake, a runtime exception, or perhaps a logic mistake? Does it position to a specific file and line variety? What module or function brought on it? These queries can guide your investigation and position you towards the accountable code.
It’s also handy to comprehend the terminology of the programming language or framework you’re employing. Error messages in languages like Python, JavaScript, or Java normally adhere to predictable styles, and Understanding to acknowledge these can significantly hasten your debugging process.
Some glitches are vague or generic, and in People cases, it’s vital to look at the context wherein the error happened. Check connected log entries, enter values, and up to date changes inside the codebase.
Don’t forget compiler or linter warnings possibly. These often precede much larger issues and provide hints about likely bugs.
In the long run, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them effectively turns chaos into clarity, aiding you pinpoint troubles a lot quicker, reduce debugging time, and become a a lot more productive and self-confident developer.
Use Logging Sensibly
Logging is One of the more effective equipment in a very developer’s debugging toolkit. When made use of correctly, it offers real-time insights into how an application behaves, helping you comprehend what’s occurring beneath the hood with no need to pause execution or phase throughout the code line by line.
A superb logging approach begins with realizing what to log and at what degree. Prevalent logging degrees include things like DEBUG, Details, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information in the course of advancement, Information for general events (like thriving start out-ups), WARN for prospective problems that don’t break the application, Mistake for genuine troubles, and FATAL when the process can’t keep on.
Stay away from flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure important messages and decelerate your technique. Give attention to important situations, condition adjustments, input/output values, and critical conclusion factors in your code.
Structure your log messages Obviously and consistently. Include things like context, including timestamps, request IDs, and performance names, so it’s simpler to trace issues in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
All through debugging, logs Allow you to track how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily useful in output environments in which stepping as a result of code isn’t achievable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Using a perfectly-believed-out logging approach, it is possible to lessen the time it will take to spot challenges, acquire further visibility into your purposes, and improve the Total maintainability and reliability of the code.
Assume Like a Detective
Debugging is not only a complex undertaking—it's a type of investigation. To properly detect and correct bugs, builders will have to method the method just like a detective resolving a secret. This state of mind will help stop working complicated concerns into manageable areas and abide by clues logically to uncover the foundation cause.
Begin by collecting evidence. Think about the symptoms of the issue: error messages, incorrect output, or functionality troubles. The same as a detective surveys against the law scene, obtain just as much applicable information and facts as you'll be able to with no jumping to conclusions. Use logs, check circumstances, and consumer reviews to piece collectively a clear picture of what’s happening.
Up coming, kind hypotheses. Question on your own: What may very well be resulting in this habits? Have any adjustments not too long ago been created for the codebase? Has this situation transpired just before under similar instances? The target will be to slim down choices and identify possible culprits.
Then, test your theories systematically. Seek to recreate the situation within a controlled ecosystem. When you suspect a particular function or part, isolate it and verify if the issue persists. Similar to a detective conducting interviews, question your code concerns and Enable the final Gustavo Woltmann coding results lead you nearer to the truth.
Pay back near interest to little aspects. Bugs typically hide from the least predicted locations—similar to a missing semicolon, an off-by-a person error, or perhaps a race ailment. Be comprehensive and affected individual, resisting the urge to patch The problem without the need of entirely understanding it. Momentary fixes might cover the real problem, only for it to resurface afterwards.
Finally, continue to keep notes on Everything you tried using and realized. Equally as detectives log their investigations, documenting your debugging procedure can help save time for long term issues and aid Many others comprehend your reasoning.
By imagining like a detective, developers can sharpen their analytical techniques, approach difficulties methodically, and come to be more effective at uncovering concealed challenges in sophisticated units.
Create Exams
Producing checks is one of the most effective strategies to help your debugging skills and Over-all enhancement efficiency. Tests not just support capture bugs early but will also function a security Web that offers you self-assurance when building variations to your codebase. A nicely-examined application is simpler to debug since it lets you pinpoint just wherever and when a dilemma occurs.
Begin with unit exams, which give attention to specific features or modules. These tiny, isolated exams can swiftly reveal regardless of whether a particular piece of logic is Functioning as anticipated. Whenever a test fails, you instantly know where to look, noticeably lessening some time expended debugging. Unit tests are Specifically beneficial for catching regression bugs—problems that reappear after Beforehand staying fastened.
Up coming, integrate integration checks and conclusion-to-conclude tests into your workflow. These aid make sure that various aspects of your software perform together effortlessly. They’re specially beneficial for catching bugs that occur in elaborate programs with numerous elements or services interacting. If a thing breaks, your exams can tell you which Component of the pipeline failed and less than what problems.
Writing assessments also forces you to Assume critically about your code. To test a attribute correctly, you would like to grasp its inputs, expected outputs, and edge situations. This level of knowledge Normally sales opportunities to better code framework and much less bugs.
When debugging a concern, writing a failing take a look at that reproduces the bug can be a strong starting point. Once the examination fails consistently, it is possible to deal with fixing the bug and look at your test move when The difficulty is resolved. This technique makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, writing exams turns debugging from the disheartening guessing sport into a structured and predictable approach—assisting you catch far more bugs, a lot quicker and much more reliably.
Just take Breaks
When debugging a tough difficulty, it’s simple to become immersed in the challenge—observing your monitor for hours, attempting Option just after Answer. But Just about the most underrated debugging equipment is actually stepping absent. Getting breaks assists you reset your intellect, reduce aggravation, and often see the issue from a new standpoint.
If you're much too near the code for much too lengthy, cognitive fatigue sets in. You may begin overlooking apparent mistakes or misreading code which you wrote just hrs earlier. Within this state, your brain gets to be much less effective at problem-solving. A brief stroll, a coffee break, or even switching to another endeavor for ten–15 minutes can refresh your concentrate. A lot of developers report discovering the root of a dilemma when they've taken time for you to disconnect, letting their subconscious work during the qualifications.
Breaks also support avoid burnout, Particularly during for a longer period debugging classes. Sitting down in front of a screen, mentally trapped, is not merely unproductive but additionally draining. Stepping absent means that you can return with renewed Vitality along with a clearer mentality. You could possibly abruptly notice a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you in advance of.
Should you’re stuck, a fantastic guideline is to established a timer—debug actively for forty five–60 minutes, then have a 5–ten minute crack. Use that time to maneuver about, stretch, or do anything unrelated to code. It may sense counterintuitive, Particularly less than tight deadlines, but it really truly leads to more rapidly and more effective debugging Eventually.
In short, using breaks is not really a sign of weak spot—it’s a wise system. It provides your Mind space to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Discover From Each individual Bug
Each and every bug you face is a lot more than just A brief setback—It can be an opportunity to develop being a developer. Whether it’s a syntax error, a logic flaw, or possibly a deep architectural challenge, every one can teach you anything precious should you make the effort to replicate and review what went Incorrect.
Commence by inquiring you a few important queries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with better practices like unit screening, code evaluations, or logging? The solutions typically reveal blind spots within your workflow or comprehension and make it easier to Make much better coding behaviors transferring ahead.
Documenting bugs can even be an outstanding practice. Retain a developer journal or keep a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see patterns—recurring challenges or prevalent faults—you can proactively prevent.
In crew environments, sharing Everything you've discovered from the bug with the peers may be especially potent. Whether it’s via a Slack concept, a short generate-up, or A fast understanding-sharing session, helping Many others stay away from the exact same difficulty boosts crew efficiency and cultivates a much better Finding out culture.
Extra importantly, viewing bugs as lessons shifts your mindset from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as essential portions of your improvement journey. In fact, several of the best builders aren't those who write best code, but those that repeatedly discover from their faults.
In the end, Just about every bug you fix adds a brand new layer on your skill set. So future time you squash a bug, take a second to replicate—you’ll come away a smarter, additional capable developer as a result of it.
Summary
Improving your debugging capabilities usually takes time, apply, and endurance — though the payoff is huge. It can make you a far more effective, self-assured, and capable developer. The following time you are knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s a chance to become superior at what you do.